08. Minkowski Sum C++
Now that you've learned the Minkowski Sum , you'll get a chance to code it in C++!
Example
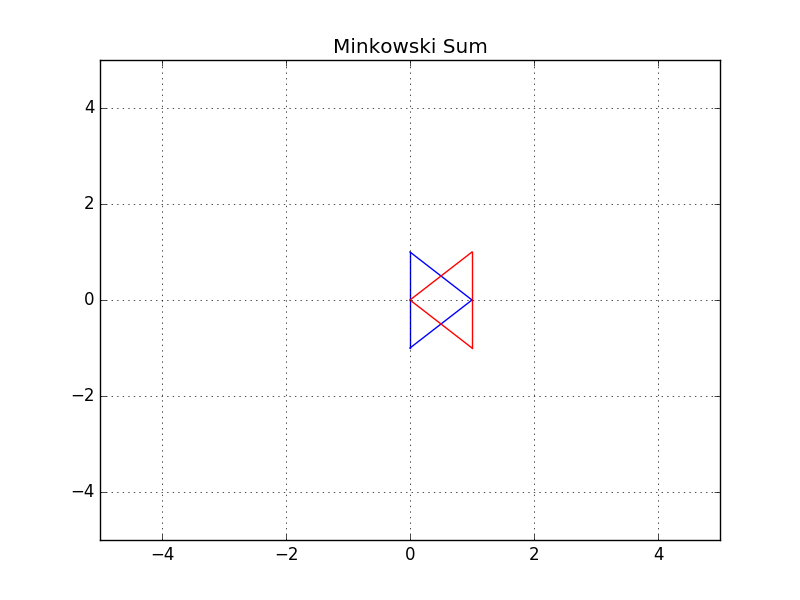
In this example, you can see two triangles - a blue and a red one. Let's suppose the robot is represented by a blue triangle and the obstacle is represented by a red triangle. Your task is to compute the configuration space C of robot A and obstacle B in C++.
- Robot : Blue triangle denoted by A
- Obstacle : Red triangle denoted by B
Minkowski Sum C++
Task Description:
Here are the steps that you should follow in order to code the Minkowski Sum in C++. Check them off as you complete them!
Task Feedback:
Great Job! Now submit your answer.
Start Quiz:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// Print 2D vectors coordinate values
void print2DVector(vector<vector<int> > vec)
{
// Sorting the vector for grading purpose
sort(vec.begin(), vec.end());
for (int i = 0; i < vec.size(); ++i) {
for (int j = 0; j < vec[0].size(); ++j) {
cout << vec[i][j] << " ";
}
cout << endl;
}
}
// ***TODO: Check for duplicate coordinates inside a 2D vector and delete them*** //
vector<vector<int> > delete_duplicate(vector<vector<int> > C)
{
}
// ***TODO: Compute the Minkowski Sum of two vectors***//
vector<vector<int> > minkowski_sum(vector<vector<int> > A, vector<vector<int> > B)
{
C = delete_duplicate(C);
return C;
}
int main()
{
// ***TODO: Define the coordinates of triangle A and B using 2D vectors*** //
// Compute the minkowski sum of triangle A and B
vector<vector<int> > C;
C = minkowski_sum(A, B);
// Print the resulting vector
print2DVector(C);
return 0;
}
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// Print 2D vectors coordinate values
void print2DVector(vector<vector<int> > vec)
{
// Sorting the vector for grading purpose
sort(vec.begin(), vec.end());
for (int i = 0; i < vec.size(); ++i) {
for (int j = 0; j < vec[0].size(); ++j) {
cout << vec[i][j] << " ";
}
cout << endl;
}
}
// Check for duplicate coordinates inside a 2D vector and delete them
vector<vector<int> > delete_duplicate(vector<vector<int> > C)
{
// Sort the C vector
sort(C.begin(), C.end());
// Initialize a non duplicated vector
vector<vector<int> > Cn;
for (int i = 0; i < C.size() - 1; i++) {
// Check if it's a duplicate coordinate
if (C[i] != C[i + 1]) {
Cn.push_back(C[i]);
}
}
Cn.push_back(C[C.size() - 1]);
return Cn;
}
// Compute the Minkowski Sum of two vectors
vector<vector<int> > minkowski_sum(vector<vector<int> > A, vector<vector<int> > B)
{
vector<vector<int> > C;
for (int i = 0; i < A.size(); i++) {
for (int j = 0; j < B.size(); j++) {
// Compute the current sum
vector<int> Ci = { A[i][0] + B[j][0], A[i][1] + B[j][1] };
// Push it to the C vector
C.push_back(Ci);
}
}
C = delete_duplicate(C);
return C;
}
int main()
{
// Define the coordinates of triangle A and B using 2D vectors
vector<vector<int> > A(3, vector<int>(2));
A = {{ 1, 0 }, { 0, 1 }, { 0, -1 },};
vector<vector<int> > B(3, vector<int>(2));
B = {{ 0, 0 }, { 1, 1 }, { 1, -1 },};
// Compute the minkowski sum of triangle A and B
vector<vector<int> > C;
C = minkowski_sum(A, B);
// Print the resulting vector
print2DVector(C);
return 0;
}
Generated Configuration Space
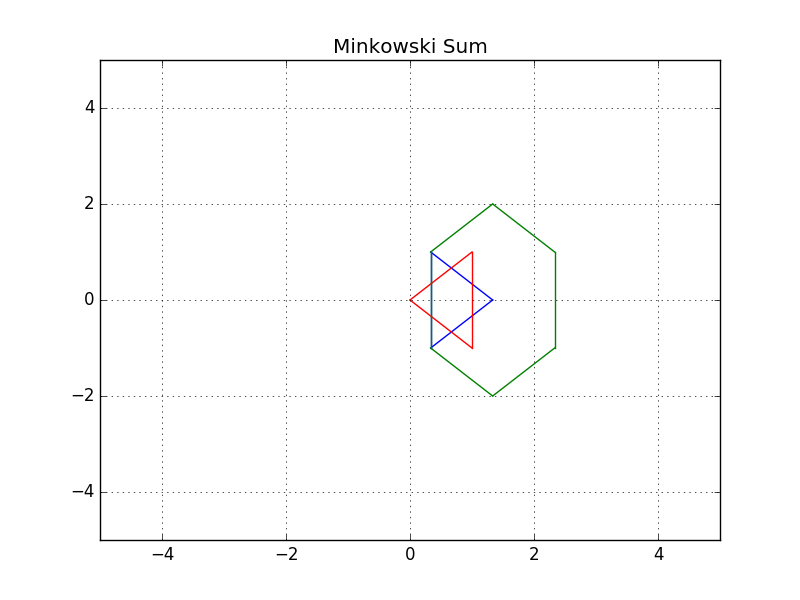
Translation
You successfully coded the Minkowski sum in C++ and generated the configuration space. You can easily notice that the red obstacle is not well inflated and the blue robot can still hit the obstacle. That's because the configuration space still has to be shifted to the obstacle.
Initially, the robot should be translated to the obstacle, and then after computing the configuration space, it should be translated to both the robot and obstacle.
Final Result
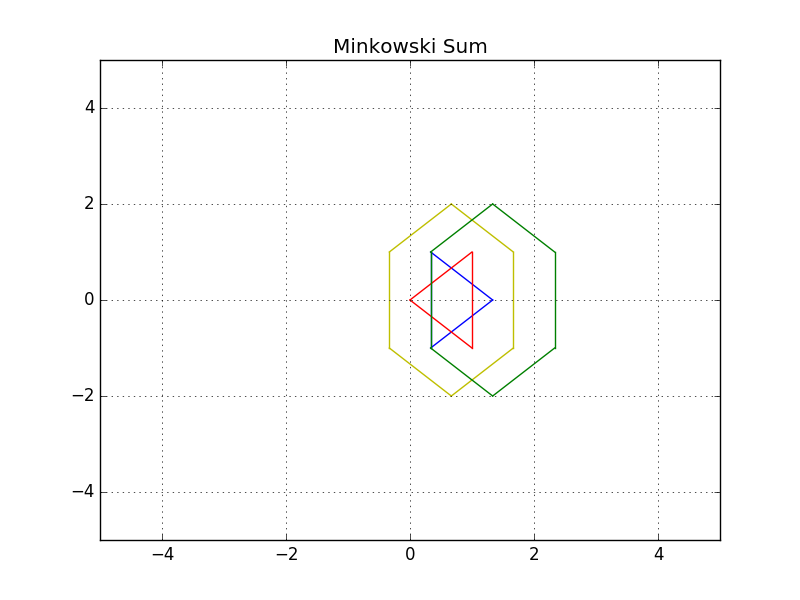
Above is the resulting image where both the blue robot and the green configuration space have been shifted. You can now see the yellow padding which represents the translated configurations space all around the red obstacle. The blue robot will never be able to hit the red obstacle since it's well inflated.
Plotting
If you are eager to know how I generated these plots and translated the shapes, you can clone the GitHub repo and read through the C++ code. In short, I had to follow these steps to generate any polygon:
- Computed the centroid of each polygon
- Computed the angle of each point-centroid with respect to the x-axis
- Sorted the points in ascending order of their angles (clockwise)
- Plotted a line between each consecutive point